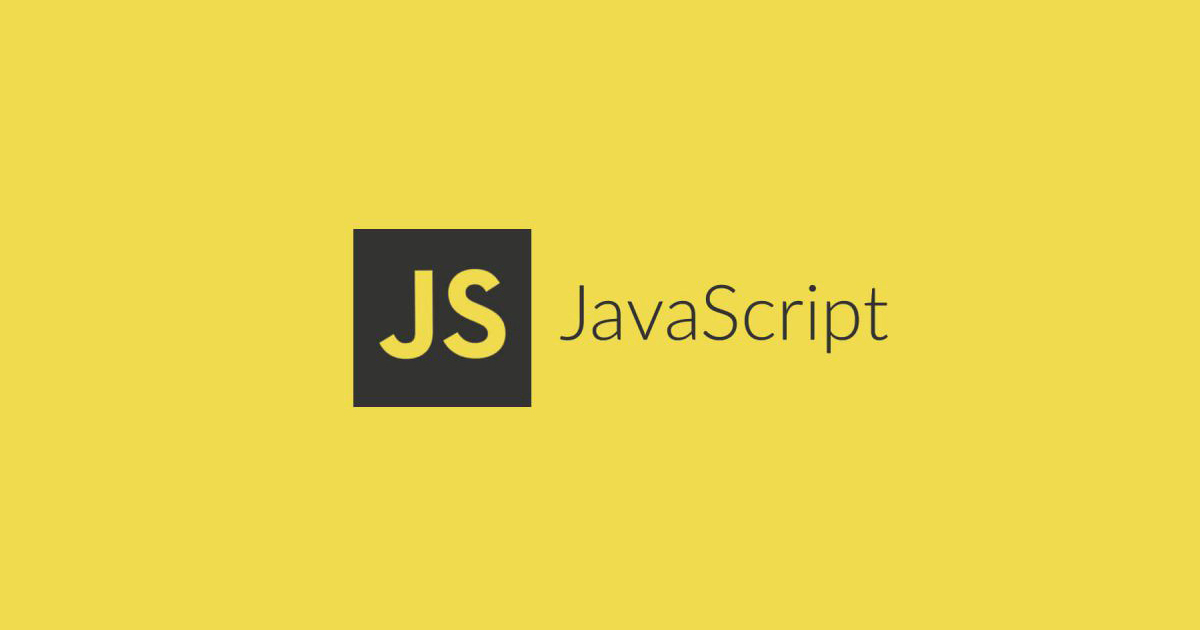
Summary
Udemy - Complete Javascript Course by Jonas Schmedtmann 강의를 듣고 각 Section별 요약을 기록.
Section 2 & 3 - JS Funcdamentals
JS 기본 문법에 대한 section.
변수 선언시 let 과 const 중 기본적으로 const 사용하는 것에 익숙해질 것. Javascript로 웹앱을 짤 때 DOM을 컨트롤하는 방식은 대부분 Event Listener를 정의, Publisher - Subscriber 패턴을 이용하므로 const로 선언. 무분별한 let 변수 사용은 코드를 지저분하게 함.
"use strict"
를 통해 strict mode activate. accidental error 등의 에러 발견을 쉽게 해주고 디버깅을 쉽게 해줌. show visible error. 항상 strict mode를 on 할 것!!
Function Declarations vs Expressions
Expression과 Declaration은 같은 방식으로 작동한다. 그러나 가장 큰 차이점은 Declaration 방식은 함수의 정의 전에 함수를 call 할 수 있고, expression 방식은 function 값을 변수에 할당하는 방식이므로 정의 이전에 call 할 경우 not defined 문제가 발생함.
그렇다면, 둘 중 어느방식이 더 좋은가? -> 본인은 Function Expression 방식을 더 선호. (Looks more structured) Javascript 에서는 function도 그냥 하나의 값이라고 생각하면 됨.
Arrow function
Function Expression 방식의 조금 더 짧은 version. Arrow function과 this
-> Arrow function에서는 자신을 둘러싸고 있는 상위 환경의 this를 그대로 계승하는 Lexical this를 따름. 그래서 Arrow function을 객체의 method
로서 정의하면 문제가 발생함. 마찬가지의 이유로 Constructor
, addEventListener의 콜백 함수
로 사용하는 것도 불가능하다.
Object
js 에서의 object는 key-value pair. 해당 key를 []를 사용하여 접근할 경우에는 key가 string이어야 함. property 이름을 명확히 아는 경우에는 dot notation을 사용, property 이름을 compute 해서 얻어야 하는 경우에는 bracket notation 사용.
const exObj = {
name: 'Dohyun',
age: 28,
hobby: 'BodyBuilding',
}
const interested = prompt("What do you want to know about exObj?);
console.log(exObj[interested]); // work well.
console.log(exObj.interested); // doesn't work.
Object method (Can’t use Arrow function)
Object의 method를 정의하는 것은 일반적인 함수 정의와 같음. 다만 function 변수가 object의 key(property) 일 뿐. this keyword 를 사용 -> Arrow function은 this나 super에 대한 바인딩이 없음. (method로 사용될 수 없다!!!)
이런식으로 method에서 새로운 property를 생성하고, 변경하는 것도 가능.
calcExFunc_Advanced: function() {
this.newProp = blahblah(calc logic) // Create new property (newProp)
return;
}
Section 8 - Behind-the-Scenes
Value types - Primitive vs Reference
Primitive type
let lastName = 'Kim';
let oldLastName = lastName;
lastName = 'Moon';
console.log(lastName);
console.log(oldLastName);
결과는 다르게 출력됨. -> String 은 Primitive value.
Reference type
const bomi = {
firstName: 'Bomi',
lastName: 'Park',
age: 28,
};
const marriedBomi = bomi;
marriedBomi.lastName = 'Kim';
console.log('Before marriage', bomi);
console.log('After marriage', marriedBomi);
결과는 똑같이 출력. -> Object는 Reference value.
그렇다면 Object는 어떻게 copy? -> Object.assign
을 이용.
const bomiCopy = Object.assign({}, bomi2); // Shallow Copy. (Only works first level)
Section 9 - Modern Operators and Strings
Enhanced Object Literals - Optional Chaining
// if( restaurant.openingHours && restaurant.openingHours.mon)
// console.log(restaruant.openingHours.mon.open);
Conosle.log(restaurant.openingHours.mon?.open);
// mon이 존재하면 open 출력. (위 두줄을 간편화)
Rest pattern and Parameters
- Destructuring
// = 기준 Right Value는 SPREAD
const arr = [1, 2, ...[3, 4]];
// = 기준 Left Value는 REST
const [a, b, ...others] = [1, 2, 3, 4, 5];
// 동시에 활용
const [pizza, salad, ...otherFoods] = [
...restaurant.mainMenu,
...restaurant.starterMenu,
];
- Functions
const add = function (...numbers) {
const reducer = (acc, cur) => acc + cur;
console.log(numbers.reduce(reducer));
};
add(2, 3);
add(5, 3, 7, 2);
const x = [23, 5, 7];
add(...x); // Spread Operator
// add function에 들어가는 순간, 다시 rest 연산자에 의해 배열로 압축됨.
// Spread operator는 배열을 요소요소로 반환.
// Rest operator는 반대로 요소요소를 배열로 반환.
- Join 2 Arrays
const wholeMenu = [...restaurant.starterMenu, ...restaurant.mainMenu];
Destructuring Object
const restaurant = {
starterMenu: ['salad', 'bread', 'soup'],
mainMenu: ['pizza', 'pasta', 'goguma'],
name: `Bonny's Pasta`,
openingHours: '9am to 8pm',
categories: ['blah', 'blahblah'],
orderDelivery({
starterIndex = 1, // default values
mainIndex = 1,
time = '19:00',
address = 'funky town',
}) {
console.log(
'Order received!',
this.starterMenu[starterIndex],
this.mainMenu[mainIndex],
time,
address
);
},
};
const deliveryObj = {
time: '22:30',
address: 'Via del Sole, 21',
mainIndex: 2,
starterIndex: 2,
};
restaurant.orderDelivery(deliveryObj);
// Object 하나만 보낸다. 그러면 fucntion에서 자체적으로 destructuring.
restaurant.orderDelivery({
mainIndex: 0,
});
const { name, openingHours, categories } = restaurant;
console.log(name, openingHours, categories);
// With new variable names
const {
name: restaurantName,
openingHours: hours,
categories: tags,
} = restaurant;
console.log(restaurantName, hours, tags);
이 외에도 Logical Operator Short Circuiting 등 여러가지 fancy한 문법이 있으나, 다른 언어에서도 활용되는 것들은 생략하였음.
Docker & Container
Docker & Container는 이 위에서 동작하는 단일 Linux Kernel을 사용하는 제어 Host가,
다수의 분리된 Linux system 을 동작시키기 위한 가상화 기법이다.
Docker는 Linux 응용 program들을 container 안에 배치시키는 것을 자동화 하는 Container 관리 SW이다.
Container Orchestration
컨테이너의 배포, 관리, 확장, networking, 가용성을 자동화해주는 도구이다.
기능 :
- provisioning 및 배포
- 설정 및 scheduling
- resource 할당
- Container 가용성 확보
- Load balancing 및 Traffic routing
- Container 상태 Monitoring
- Container 간 상호 작용의 보안 유지
ex) Kubernetes, Docker Swarm, Apache Mesos
Object
- POD : Kubernetes 의 가장 기본적인 배포 단위로, Container를 포함하는 단위.
-> Kubernetes는 하나의 Container를 개별적으로 하나씩 배포하는 것이 아니라, POD라는 단위로 배포하며, POD는 하나 이상의 Container를 포함. - Service : Kubernetes에서 변하지 않는 IP주소와 port를 제공.
-> Kubernetes에서 POD는 일회성이라 언제든지 이동하거나 사라질 수 있어, POD를 연결하기 위한 단일 진입점 역할을 해 준다. - Volume : Container의 외장 디스크
-> POD가 시작할 때 Container마다 Local disk를 생성하여 시작되는데, 이러한 Local disk의 경우 Container가 종료되면 기록된 내용이 유실되어 영구적으로 내용을 저장하고자 할 때 이용. - Namespace : 한 Kubernetes cluster 내의 논리적인 분리 단위.
Docker & Kubernetes 설치 및 환경구축.
다음 내용들은 회사의 vdi (CentOS7) 가상 머신 두 대를 이용하였음.
(한 대는 Master node, 다른 한 대는 Worker node 로 구성할 것임.)
(root 계정으로 접속을 가정.)
이 중 277번 Machine 이 Master node, 278번 Machine이 Worker node로 설정하였음
-
Docker 설치
install requirements
sudo apt-get install \ apt-transport-https \ ca-certificates \ curl \ gnupg-agent \ software-properties-common
Add Docker repository
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo apt-key add - sudo apt-key fingerprint 0EBFCD88 sudo add-apt-repository \ "deb [arch=amd64] https://download.docker.com/linux/ubuntu \ $(lsb_release -cs) \ stable"
Install Docker
sudo apt-get update sudo apt-get install docker-ce docker-ce-cli containerd.io
확인
systemctl enable docker && systemctl start docker
docker version
-
Kubernetes Apt Repository 구성
sudo apt-get install -y apt-transport-https curl curl -s https://packages.cloud.google.com/apt/doc/apt-key.gpg | sudo apt-key add - cat <<EOF | sudo tee /etc/apt/sources.list.d/kubernetes.list deb https://apt.kubernetes.io/ kubernetes-xenial main EOF sudo apt-get update
- 통신 문제를 방지하기 위한 SE Linux 모드 변경
setenforce 0
-
Kubernetes 설치 & Kubelet 활성화 (k8s는 안정된 version인 1.15.5 로 설치를 권장)
sudo apt-get install -y kubelet=1.15.5-00 kubeadm=1.15.5-00 kubectl=1.15.5-00 systemctl enable kubelet && systemctl start kubelet
-
자동 업데이트 방지
sudo apt-mark hold kubelet kubeadm kubectl
-
IPTables(방화벽 설정하는 도구) 설정
(/proc/sys/net/ipv4/ip_forward
를 1 로 설정하는 작업)vi /etc/sysctl.conf net.bridge.bridge-nf-call-ip6tables = 1 net.bridge.bridge-nf-call-iptables = 1 net.ipv4.ip_forward = 1
-
IPTables 설정 파일 적용
sysctl --system
-
br_netfileter 모듈 load
lsmod | grep br_netfilter
- Port 허용
firewall-cmd --permanent --add-port=6443/tcp firewall-cmd --permanent --add-port=10250/tcp firewall-cmd --reload
-
Kubernetes 초기화에 사용될 이미지 pull
kubeadm config images pull
-
Swap error처리
swapoff -a
, 또한vi /etc/fstab
에서 swap 설정 주석처리 -> 영구적으로 끔. -
Kubernets Cluster 설정(Master Only)
kubeadm init --pod-network-cidr=10.244.0.0/16
- Kubernetes Cluster 접근 설정(Master Only)
mkdir -p $HOME/.kube cp -i /etc/kubernetes/admin.conf $HOME/.kube/config chown $(id -u):$(id -g) $HOME/.kube/config
- Kube flannel 적용(Master Only)
wget https://raw.githubusercontent.com/coreos/flannel/v0.9.1/Documentation/kube-flannel.yml ip a # Network Interface 명 확인 vi ube-flannel.yml # command에 --iface=network-interface명 추가.
- CNI(Container Network Interface) 설치(Master Only)
curl https://docs.projectcalico.org/archive/v3.8/manifests/calico.yaml -O kubectl apply -f calico.yaml kubectl apply -f kube-flannel.yml
- Kubernetes 주요 POD 동작 확인 & master node 연결 확인(Master Only)
kubectl get pod -n kube-system kubectl get nodes
(calico 관련 오류 - 방화벽 문제이므로 systemctl stop firewalld
로 방화벽 아예 꺼보자.)
여기까지 성공하였다면, Master node 구성 완료.
이제 Master node 에 Worker node를 붙여 보자.
-
Master node에 추가(Worker Only)
kubeadm join [master-ip]:6443 --token [token] --discovery-token-ca-cert-hash sha256:[hash]
- Token & Hash값 확인(Master node에서 확인)
kubeadm token list openssl x509 -pubkey -in /etc/kubernetes/pki/ca.crt | openssl rsa -pubin -outform der 2>/dev/null | \ openssl dgst -sha256 -hex | sed 's/^.* //'
- Token list에 값이 없을 경우 Token 생성
kubeadm token create
결과 확인.
참고. Kubernetes master IP가 바뀌었을때
git clone https://github.com/dohyunKim12/kubernetes.git